I looked at the docs and tried to modify my code as you suggested. However, now the same problem I was running into with marked is happening with showdown too where my Previewer and Editor aren’t rendering at all (leading to less tests passing). (the code I had earlier had the Editor and Previewer rendering properly and it did convert the line breaks into html properly from markdown language)
link to my codepen again: https://codepen.io/cmalloy/pen/rNPyNeG
previous code for reference (though I’m starting to think the issue is how codepen loads the libraries though I might be wrong):
const defaultMarkdown = `# Your Main Header Here
## Your subheading
### Another kind of heading
Here is an example of how to write \`code\` in an inline with backticks.
\`\`\`
// This is a multiline code block
exampleArray = [
{ foodType: "pie", flavor: "apple" },
{ foodType: "ramen", flavor: "chicken" },
{ foodType: "oatmeal", flavor: "apple cinnamon" }
];
\`\`\`
;
const Editor = ({ markdownText, onInputChange }) => {
return (
<div id="editor-container" className="editor-container">
<div id="editor-toolbar" className="editor-toolbar">
<span><i className="fa-solid fa-pen-nib"></i> Editor</span>
<i className="fa-solid fa-maximize"></i>
</div>
<textarea
id="editor"
className="editor"
type="text"
defaultValue={markdownText}
onChange={onInputChange} // Use the passed onInputChange function
/>
</div>
);
};
const markdownConverter = new showdown.Converter();
const Previewer = ({ markdownText }) => {
const htmlOutput = markdownConverter.makeHtml(markdownText);
return (
<div id="preview-container" className="preview-container">
<div className="preview-toolbar">
<span><i className="fa-solid fa-eye"></i> Preview</span>
<i className="fa-solid fa-maximize"></i>
</div>
<div id="preview" className="preview" dangerouslySetInnerHTML={{ __html: htmlOutput }}></div>
</div>
);
};
const App = () => {
const [markdownText, setMarkdownText] = React.useState(defaultMarkdown);
const handleInputChange = (event) => {
setMarkdownText(event.target.value);
};
return (
<div id="outer-container">
<Editor markdownText={markdownText} onInputChange={handleInputChange} />
<Previewer markdownText={markdownText} />
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
current javascript code (it has this error despite having showdown externally rendered: Uncaught ReferenceError: Showdown is not defined):
const App = () => {
const [markdownText, setMarkdownText] = React.useState(defaultMarkdown);
const initializeMarkdown = () => {
const showdown = new Showdown.Converter({
tables: true,
strikethrough: true,
tasklists: true,
smoothLivePreview: true,
});
const defaultMarkdown = `# Your Main Header Here
## Your subheading
### Another kind of heading
Here is an example of how to write \`code\` in an inline with backticks.
\`\`\`
// This is a multiline code block
exampleArray = [
{ foodType: "pie", flavor: "apple" },
{ foodType: "ramen", flavor: "chicken" },
{ foodType: "oatmeal", flavor: "apple cinnamon" }
];
\`\`\`
- This is a list item
- Another list item
> This is a blockquote
Here is an 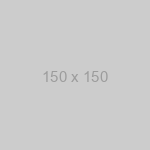 and some **bold text**.
This is a [link](https://www.example.com).
And some \`inline code\`.
`;
const Editor = ({ markdownText, onInputChange }) => {
// ... (unchanged)
};
const Previewer = ({ markdownText }) => {
const htmlOutput = showdown.makeHtml(markdownText);
return (
<div id="preview-container" className="preview-container">
{/* ... (unchanged) */}
</div>
);
};
const handleInputChange = (event) => {
setMarkdownText(event.target.value);
};
ReactDOM.render(
<div id="outer-container">
<Editor markdownText={markdownText} onInputChange={handleInputChange} />
<Previewer markdownText={markdownText} />
</div>,
document.getElementById("root")
);
};
React.useEffect(() => {
initializeMarkdown();
}, []);
return null;
};
\`\`\`
ReactDOM.render(<App />, document.getElementById("root"));
Scripts externally rendered(in the order they’re being rendered):
https://cdn.freecodecamp.org/testable-projects-fcc/v1/bundle.js
https://unpkg.com/react@16.13.1/umd/react.development.js
https://unpkg.com/react-dom@16.13.1/umd/react-dom.development.js
https://cdnjs.cloudflare.com/ajax/libs/showdown/1.10.2/showdown.min.js
(I also tried replacing the cdn with and unpkg and got the same error)